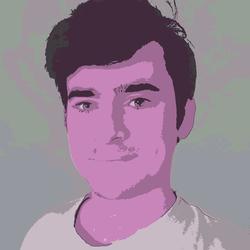
Python bug/feature which costed me an hour of my life
What's wrong with the code below?
This function :
>>> def func(n=5, arr=[]):
... arr.extend(["hi"] * n)
... return arr
- Takes in integer value, n and an array, arr
- Appends n "hi"s to the array
- returns the resulted array
>>> func(3)
['hi', 'hi', 'hi']
But... what if we call it again?
>>> func(3)
['hi', 'hi', 'hi', 'hi', 'hi', 'hi']
Wait, what? n is 3, so why do we get 6 "hi"-s ?
>>> func(5)
['hi', 'hi', 'hi', 'hi', 'hi', 'hi', 'hi', 'hi', 'hi', 'hi', 'hi']
huh? 13 times? Why does it append to the previous result??
Well, after playing with various iterations, I came to realize that the default value inside the function definition is created only once.
I.e. inside the def func(n=5, arr=[]):
python remembers to use created empty array as a default value. But actually, it only remembers the reference to the array, not the array itself. So when it's modified, still in the next call it still refers to the same object, it uses the modified array as a default.
Anyway, that's kinda insane and cool, but I've read on StackOverflow that it has some good reasons. I'll post a link later, gotta take a shower.